To develop an app that allows users to log in, create a profile, link their X (formerly Twitter) account, and display their tweets, you’ll need to follow a clear roadmap that integrates the X API v2 for fetching tweets. Here’s how to approach it:
1. Set Up X Developer Account & API Access
- Sign Up and Create a Developer Account:
- Go to the X Developer Portal and register as a developer.
- Create a project and an app within the developer portal.
- Get API Keys and Tokens:
- Under the App Settings in the Developer Portal, retrieve the following credentials:
- API Key
- API Secret Key
- Bearer Token
- Access Token and Access Token Secret (if needed for user authentication)
- Under the App Settings in the Developer Portal, retrieve the following credentials:
- Set Up App Permissions:
- In the App settings, ensure permissions are set to Read and Write (if users need to post tweets later).
- Enable OAuth 2.0 for user authentication.
2. Set Up Backend for OAuth 2.0 Authentication
You need to let users log in to their X account securely using OAuth. Follow these steps:
- Install Libraries for OAuth: Use a library for OAuth 2.0 in your backend. Examples:
- Python:
requests-oauthlib
- Node.js:
passport-twitter
ortwitter-api-v2
- PHP:
league/oauth2-twitter
- Python:
- Redirect User to X for Authentication:
- Use the OAuth 2.0 Authorization Code Flow:
- Redirect users to the X authorization URL.
- After successful login, X will redirect users back to your app with an authorization code.
- Exchange the authorization code for an access token.
https://api.twitter.com/oauth2/authorize?response_type=code&client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&scope=read,tweet.read,users.read
- Use the OAuth 2.0 Authorization Code Flow:
- Store Tokens: After retrieving the access token and refresh token, securely store them in your database, associated with the user’s profile.
3. Fetch User Tweets
After the user has linked their X account, you can fetch their tweets using the X API:
- API Endpoint for Fetching Tweets: Use the X API v2 endpoint to fetch user tweets:
GET https://api.twitter.com/2/users/:id/tweets
- Steps:
- Retrieve the user’s X account ID using the
GET /2/users/me
endpoint. - Use the returned
user_id
to fetch their tweets.
curl -X GET \ 'https://api.twitter.com/2/users/{user_id}/tweets' \ -H 'Authorization: Bearer YOUR_ACCESS_TOKEN'
- Retrieve the user’s X account ID using the
- Display Tweets in Your App: Parse the response and display the tweets on the user’s profile page.
4. Build the App Frontend
Your app frontend should include:
- Login & Profile Creation:
- Use traditional methods (email/password or third-party login like Google).
- Store user details in your database.
- Link X Account:
- Add a “Link X Account” button.
- On click, redirect the user to the X OAuth authorization page.
- Display Tweets:
- Fetch tweets via the backend and render them dynamically on the user’s profile.
5. Handle Usage Limits
The X API v2 has usage caps, so:
- Read: Make sure you don’t exceed the limit of 100 tweets per month (as shown in your screenshot). You might need to request elevated access if required.
- Cache responses in your database to minimize repeated API calls.
6. Security and Best Practices
- Secure API Keys:
- Never expose your API keys or tokens on the frontend. Use a secure backend to interact with the API.
- Protect User Data:
- Encrypt access tokens and sensitive data.
- Rate Limiting and Caching:
- Implement rate-limiting logic to avoid exceeding API caps.
- Cache tweets to reduce API calls.
Example Workflow:
- User logs in to your app.
- User clicks “Link X Account” and authenticates via X OAuth.
- Your app retrieves the access token and uses it to fetch the user’s tweets.
- Display the tweets on the user’s profile page.
If you need code samples or more guidance for a specific step (e.g., OAuth implementation), let me know! 😊
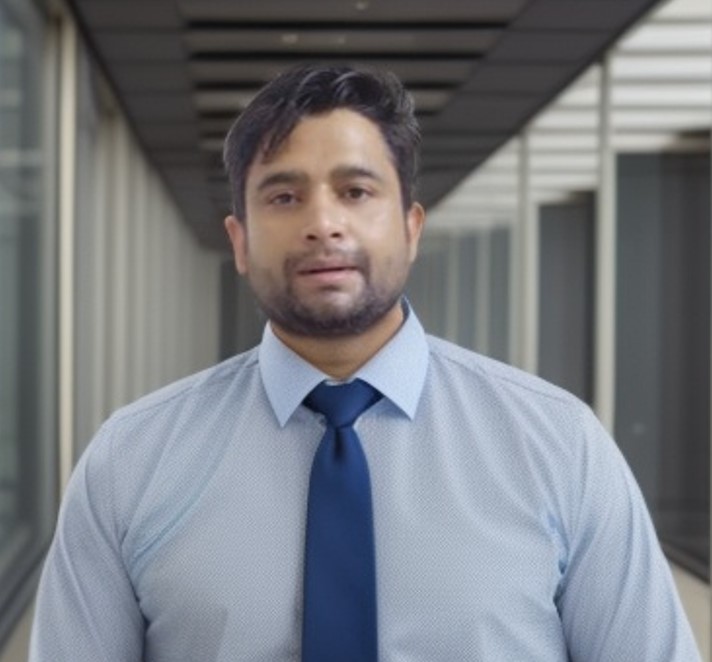
I’m a DevOps/SRE/DevSecOps/Cloud Expert passionate about sharing knowledge and experiences. I am working at Cotocus. I blog tech insights at DevOps School, travel stories at Holiday Landmark, stock market tips at Stocks Mantra, health and fitness guidance at My Medic Plus, product reviews at I reviewed , and SEO strategies at Wizbrand.
Please find my social handles as below;
Rajesh Kumar Personal Website
Rajesh Kumar at YOUTUBE
Rajesh Kumar at INSTAGRAM
Rajesh Kumar at X
Rajesh Kumar at FACEBOOK
Rajesh Kumar at LINKEDIN
Rajesh Kumar at PINTEREST
Rajesh Kumar at QUORA
Rajesh Kumar at WIZBRAND